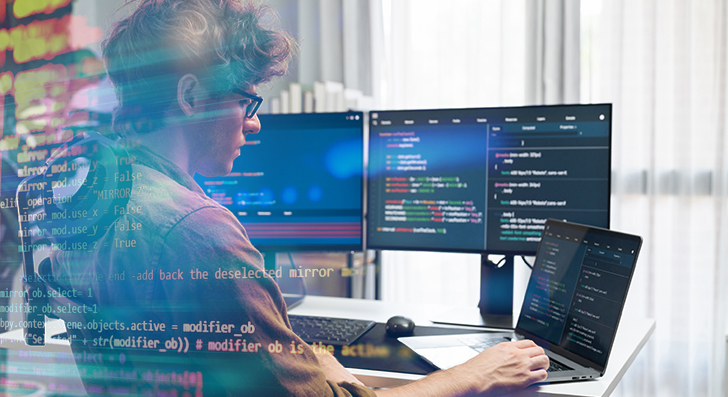
Scalability suggests your software can take care of development—much more buyers, additional knowledge, and a lot more site visitors—with out breaking. Like a developer, developing with scalability in your mind saves time and worry later on. Right here’s a transparent and useful guide that will help you get started by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be part of your respective strategy from the beginning. Quite a few applications fall short once they improve quick for the reason that the original structure can’t cope with the extra load. Being a developer, you need to Consider early regarding how your method will behave stressed.
Start by planning your architecture to generally be adaptable. Avoid monolithic codebases the place everything is tightly connected. As a substitute, use modular design or microservices. These designs split your application into more compact, unbiased elements. Just about every module or service can scale on its own devoid of affecting The entire process.
Also, think about your databases from working day 1. Will it need to have to take care of one million customers or maybe 100? Pick the ideal variety—relational or NoSQL—dependant on how your data will expand. Approach for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A further important stage is to stop hardcoding assumptions. Don’t generate code that only works under present situations. Think of what would come about If the person foundation doubled tomorrow. Would your application crash? Would the database decelerate?
Use design styles that aid scaling, like information queues or party-pushed units. These assistance your app deal with much more requests without having acquiring overloaded.
Once you Construct with scalability in mind, you are not just making ready for fulfillment—you might be lessening upcoming complications. A properly-planned method is easier to take care of, adapt, and improve. It’s greater to organize early than to rebuild later on.
Use the correct Database
Deciding on the appropriate database is a vital Component of constructing scalable applications. Not all databases are constructed the identical, and using the Erroneous one can slow you down or simply lead to failures as your app grows.
Get started by comprehension your info. Could it be highly structured, like rows in a very table? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are definitely solid with associations, transactions, and consistency. They also assistance scaling procedures like read through replicas, indexing, and partitioning to handle extra website traffic and info.
If your details is much more flexible—like consumer activity logs, merchandise catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling significant volumes of unstructured or semi-structured info and will scale horizontally far more effortlessly.
Also, look at your go through and generate patterns. Are you presently carrying out many reads with less writes? Use caching and browse replicas. Are you handling a hefty publish load? Look into databases that will cope with higher publish throughput, or even occasion-dependent details storage methods like Apache Kafka (for temporary information streams).
It’s also clever to Imagine ahead. You may not want State-of-the-art scaling options now, but choosing a database that supports them implies you gained’t have to have to modify later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your knowledge determined by your entry styles. And generally observe database efficiency while you increase.
Briefly, the appropriate databases relies on your app’s structure, velocity needs, And exactly how you count on it to expand. Get time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Speedy code is essential to scalability. As your app grows, each and every smaller hold off adds up. Poorly created code or unoptimized queries can decelerate general performance and overload your procedure. That’s why it’s essential to Make productive logic from the start.
Start by creating thoroughly clean, easy code. Avoid repeating logic and take away everything unneeded. Don’t choose the most complex Option if an easy 1 is effective. Keep the capabilities limited, focused, and straightforward to test. Use profiling tools to uncover bottlenecks—spots exactly where your code takes way too lengthy to operate or takes advantage of an excessive amount memory.
Up coming, look at your database queries. These often sluggish things down much more than the code by itself. Be certain Every single question only asks for the information you actually will need. Steer clear of Pick out *, which fetches every little thing, and in its place pick precise fields. Use indexes to speed up lookups. And prevent doing too many joins, Primarily across massive tables.
In case you see the identical facts becoming requested time and again, use caching. Shop the outcome quickly using equipment like Redis or Memcached therefore you don’t have to repeat highly-priced operations.
Also, batch your database operations any time you can. Rather than updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with substantial datasets. Code and queries that perform high-quality with a hundred documents might crash once they have to deal with 1 million.
In a nutshell, scalable applications are speedy applications. Keep the code limited, your queries lean, and use caching when needed. These actions enable your software keep clean and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to manage additional buyers plus more traffic. If everything goes via one particular server, it is going to speedily turn into a bottleneck. That’s the place load balancing and caching are available in. These two tools help keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across several servers. In place of just one server undertaking every one of the perform, the load balancer routes customers to various servers based on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing info temporarily so it could be reused swiftly. When users ask for the identical info all over again—like a product page or maybe a profile—you don’t ought to fetch it in the databases anytime. You'll be able to provide it through the cache.
There are two prevalent varieties of caching:
one. Server-aspect caching (like Redis or Memcached) suppliers knowledge in memory for fast entry.
2. Customer-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances speed, and can make your application a lot more economical.
Use caching for things that don’t transform frequently. And normally ensure your cache is updated when knowledge does change.
In a nutshell, load balancing and caching are very simple but effective instruments. Together, they help your application handle a lot more people, stay quickly, and Get well from problems. If you intend to grow, you will need both equally.
Use Cloud and Container Tools
To website construct scalable apps, you would like tools that let your app increase conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling Significantly smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t should invest in components or guess upcoming capacity. When traffic raises, you'll be able to incorporate far more assets with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability instruments. You may center on making your application as opposed to handling infrastructure.
Containers are An additional important Instrument. A container deals your app and everything it really should operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app concerning environments, from the laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application takes advantage of many containers, equipment like Kubernetes assist you to regulate them. Kubernetes handles deployment, scaling, and Restoration. If 1 section of your respective app crashes, it restarts it automatically.
Containers also help it become simple to different areas of your app into expert services. You'll be able to update or scale parts independently, and that is great for overall performance and trustworthiness.
In a nutshell, using cloud and container instruments indicates you may scale quickly, deploy conveniently, and Recuperate immediately when difficulties materialize. If you'd like your application to develop without the need of limits, start out utilizing these equipment early. They save time, minimize danger, and make it easier to stay focused on making, not correcting.
Check Anything
In the event you don’t keep an eye on your software, you won’t know when issues go Mistaken. Checking helps you see how your app is undertaking, spot concerns early, and make superior conclusions as your app grows. It’s a critical Element of developing scalable techniques.
Start out by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this knowledge.
Don’t just keep an eye on your servers—watch your application much too. Regulate how long it takes for consumers to load web pages, how frequently problems come about, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Put in place alerts for significant challenges. One example is, If the reaction time goes previously mentioned a limit or even a support goes down, you ought to get notified instantly. This assists you repair issues fast, frequently prior to users even see.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in errors or slowdowns, you could roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and knowledge boost. Without checking, you’ll skip indications of issues right up until it’s as well late. But with the ideal equipment in place, you keep in control.
Briefly, monitoring can help you maintain your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about understanding your technique and making sure it really works well, even stressed.
Final Ideas
Scalability isn’t only for huge companies. Even modest applications need to have a solid Basis. By creating thoroughly, optimizing wisely, and utilizing the ideal resources, you could Develop applications that grow easily devoid of breaking under pressure. Start off compact, Believe major, and build wise.